Computing Coursework
Database Concepts
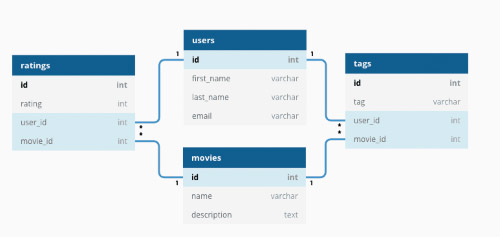
This was an introductory level course that taught the basics about the Relational Database Model and using SQL to create and manipulate databases.
In the first half of the semester, I learned the relational database model and how to normalize data to the third normal form. We then learned how to create Entity Relationship Diagrams with Crows Feet Notation, which would eventually be used to create databases.
In the second half of the semester, we used Structured Query Language (SQL) to create and manipulate databases. We learned about the difference between Data Definition Language (DDL) and Data Manipulation Language (DML) as well as the basic SQL commands for DDL and DML operations. We also learned the aggregation functions and briefly discussed some more advanced concepts, such as indices, triggers, transactions, etc.
For my final project in this class, I created a database for storing information for a video game retailer like GameStop. First, I decided on and identified the attributes for the four distinct entities in this database: games, employees, customers, and transactions. Once I decided what data I would include in the database, I then created an ERD using an online drawing program that highlighted the relationships between each of the entities. Then, I created the database in SQL using hundreds of rows of randomly generated data for each entity. Finally, I decided upon 8 different queries that I would use to retrieve certain data, each one increasing in difficulty, and I wrote SQL to execute these queries.
Object Oriented Programming
In this introductory level programming course, I learned the basics of general programming and how to implement object-oriented programming with Java and JavaFX.
In the beginning of the semester, we learned the basics of general programming, including variables, data types, using built-in classes and methods, conditional statements, and loops. We were encouraged to explore the Java API to find methods that could allow us to implement solutions for our in-class exercises and homework problems.
In the later part of the class, we learned about some of the major principles of Object-Oriented Programming, including inheritance and encapsulation. We learned how to build object classes from scratch and created driver classes that allowed us to test the objects we created. We also used JavaFX, which is a collection of packages that allows users to create Graphical User Interfaces (GUIs). For the final project of the class, I created a dynamic visualization that displayed inmate data for the Allegheny County jail system. This visualization was a bar chart that tracked the number of incarcerations on a given day. Additionally, I implemented drop-down bars that allowed the user to display subsets of the data based on gender and/or race as well as two search fields that limited the data based on minimum and maximum age.
Digital Media
In the first half of this class, we learned about the basic design principles as well as the differences between designing for print and for the web. We also learned how to use Photoshop to digitally manipulate images. We learned how to use the Camera Raw Filter to adjust the lighting of images. Then, we learned how to apply a variety of effects to images, such as motion blurs, oil painting effects, polaroid filters, and a plethora of other methods. For the culminating project of this part of the term, we created a portfolio of five images that were manipulated in photoshop. In addition to producing quality photos, I wrote a 250-word description for each image detailing the decisions that I made when creating the photo and how these decisions increased the quality of the image.
In the second half of the term, I learned how to conduct a social media assessment like those done at large companies on a quarterly, semi-annual, or annual basis. I created a social media portfolio that highlighted the major goals and objectives that the CIS department should pursue. I identified the different constituencies that would be viewing our media and how we would appeal to each constituency. I also made an argument for establishing a presence on Instagram and provided five example images and captions for posts that go on the Instagram page. I also used photoshop to create a logo for the Instagram page. I ended the report with a reflection section that discussed what things were done in the past that did not work or no longer work and how to address each of these issues so that the goals and objectives identified at the beginning of the report will be met.
Introduction to Web Design & Development
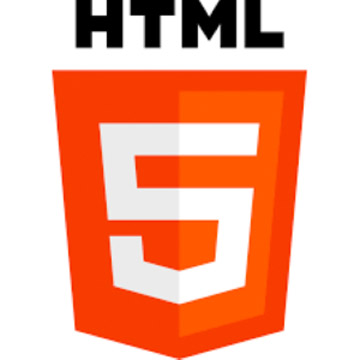
This was an introductory course in Web Development that taught me how to use HTML and CSS as well as how to implement design principles to create effective web content.
First, we learned about the history of the web to develop an understand of how we eventually reached HTML5. I then learned how to write HTML code to develop the basic structure of a site. I completed a variety of exercises which forced me to use a variety of different HTML elements, including anchors (hyperlinks), tables, lists, etc. I also learned about DIVs and the structural elements in preparation for CSS.
Later in the term, we learned how to use CSS. At first, we used CSS to control color schemes and typography. Eventually, we learned how to use CSS to do basic layout manipulation, such as creating multi-column layouts. At the end of the term, we learned how to create fully responsive web content by implementing media queries that changed the layout of websites for different sized devices.
The final project for this class was to build this website. Along with creating this website, I also had to write a description of the choices I made when designing this site and how those choices created an effective design.
Simulations
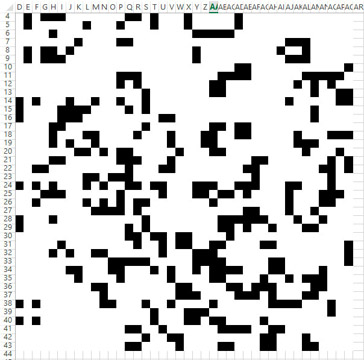
In this advanced data analysis course, we learned how to design, code, and test computer-based simulations. We spent the majority of the semester learning about different types of simulations, including deterministic models, which produce the same results with every run, and stochastic models, which use an element of randomness to produce different results upon each run. We also learned about some of the tools that are commonly used in stochastic simulations, including Markov Chains, Hidden Markov Models, Monte Carlo Sampling, and Markov Chain Monte Carlo (MCMC).
The first major project I completed for this class was done individually, and I created a simulation that extended Conway’s Game of Life. The Game of Life, which is pictured above, is a cellular automaton played on a grid where each square on the grid is either alive or dead. There are a set of rules that determine whether a cell becomes alive or dead in the next iteration of the simulation based on the state of its neighbors. For this assignment, I had to add a 3x3 predator to the game of life that could consume live cells. This “predator” traveled either horizontally or vertically across the grid and consumed energy when moving but gained energy upon consumption of living cells. Once a predator ran out of energy, it would “die” and turn into a mass of live cells. I also implemented a Markov chain that gave predators a random chance of reproducing given they met an energy threshold for reproduction.
The second project I did for this class was in conjunction with two other students. We created a deterministic simulation from scratch that attempted to predict the optimal “break” in a game of 9 ball pool. First, we created an agent-based simulation where each ball on the pool board was treated as a separate object. Then, we created a ranking algorithm that scored a break according to how likely the player was to win the game if they continued the game from the position after the break. Finally, we ran a simulation for starting the cue ball at a variety of different positions and generated a heat map that showed the scores of the ranking algorithm for a break and its corresponding initial position. We used the results of this simulation to generate strategies for the game of 9 ball pool.
Networks
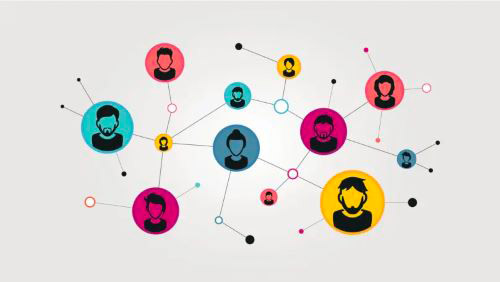
This was another advanced data analytics course where I learned how to analyze the structure of networks in Python, specifically using the JupyterLab environment. In this class, we primarily used the NetworkX library in Python, which treated networks as graphs, objects which can be analyzed more deeply with Graph Theory.
In this class, we learned about different types of networks, including undirected networks where edges no direction, directed networks in which edges are directed from one node to another, multipartite networks where nodes can be separated into different groups, and multilayer networks which are used to represent different types of edges, often ones that exist during different periods of time. We learned about different metrics and methods that can be used to describe a network, including community detection, locating important nodes, and assessing density. We also learned methods for predictive network analysis, including how information and disease may spread through social networks.
I completed three main projects for the class. The first of these projects was an assignment where I created both a static and dynamic visualization of a power gird network, each of which revealed different information about the underlying network. The second project was a descriptive network analysis assignment where I looked at a social network of interactions in the Marvel Comic Universe. I compared the insights generated from the analysis to the descriptions of superheroes on marvel.com to see if the results were consistent with the true Marvel universe. For the final project in this class, I used both descriptive and predictive methods to analyze a social network of Donald Trump. First, I use common metrics to assess whether the Trump network has the same characteristics as common social networks. Then, I found which people the most influential in this network, which unsurprisingly included the Trump family. Finally, I assessed how quickly information could cascade through the network if a new political idea originated from Trump and a few of his close relatives and associates.